2. Getting your API key
Getting your Bond API identity and authorization keys.
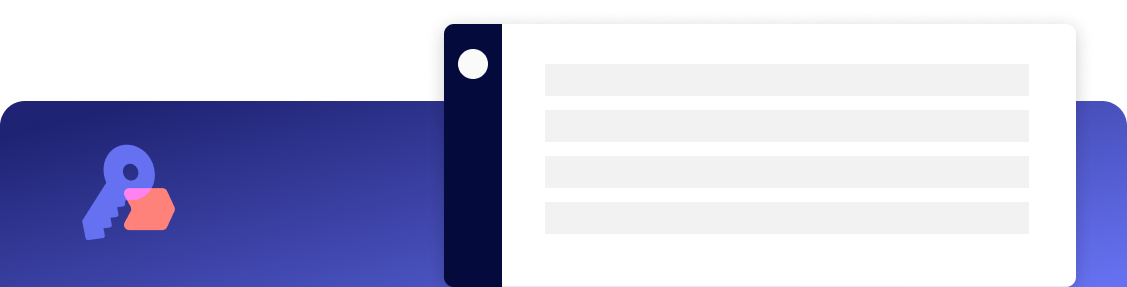
First, you'll need to Sign up for Bond and then use the login provided to log in.
What is your API key used for?
An API key is used in requests from your Bond account to the Bond platform APIs. API keys are associated with your Bond account and not with individual user accounts that generate the keys. When you generate an API key, all members of your Bond account can use it.
You can use the same API key to access all BOND Studio APIs. For incoming requests, Bond APIs require authentication using both the identity and authorization parts of an API key in the request header. The authorization is confidential to your brand and is used to verify your identity.
Your brand can have any number of API keys, each with a varying levels of permissions. Currently, all API keys generated have access to all endpoints within the Bond platform.
API key | Example |
---|---|
Identity | fg6760e3-5af8-432a-9072-bbd4072d23e9 |
Authorization | MLF0su9R5m9cpaOSRk/vY8/VqCVGWy/AV/qdZMPG/6s58beK6WKyZ8D4W6jemiUY |
Perform the steps below to get a sandbox API key. You can also request a production key, but this is not enabled until the integration with your bank partner is complete.
- Log in to the Bond Portal management app.
- Go to Developers > API Keys.
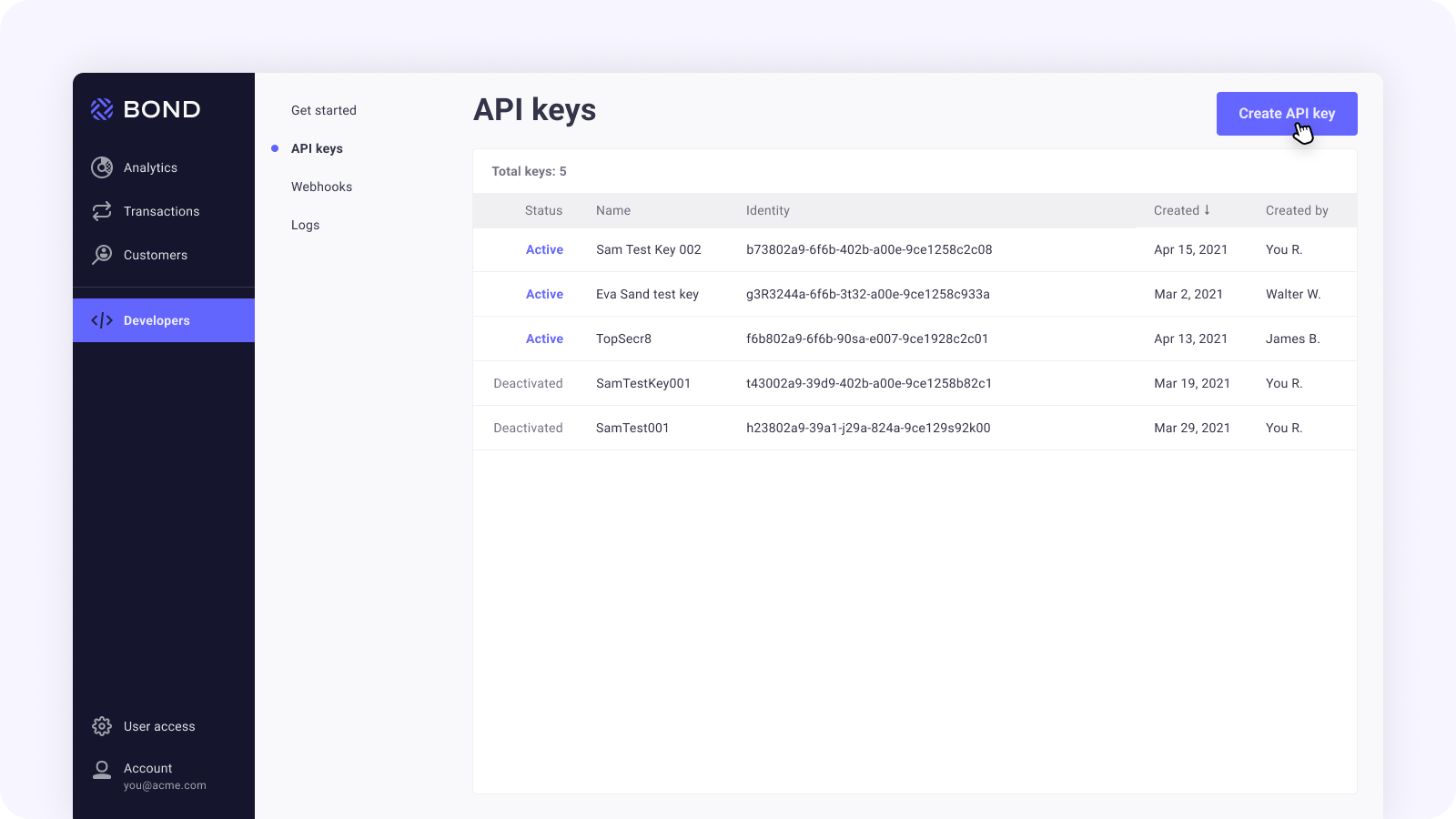
- Click Create API key.
- Enter the name you want for the new API key and click Create key.
Naming restrictions
- At least three characters
- No more than 128 characters
- Must not contain spaces
- Must not contain any of the following characters; / ^ ( ? ! \ s + $ ) . * /
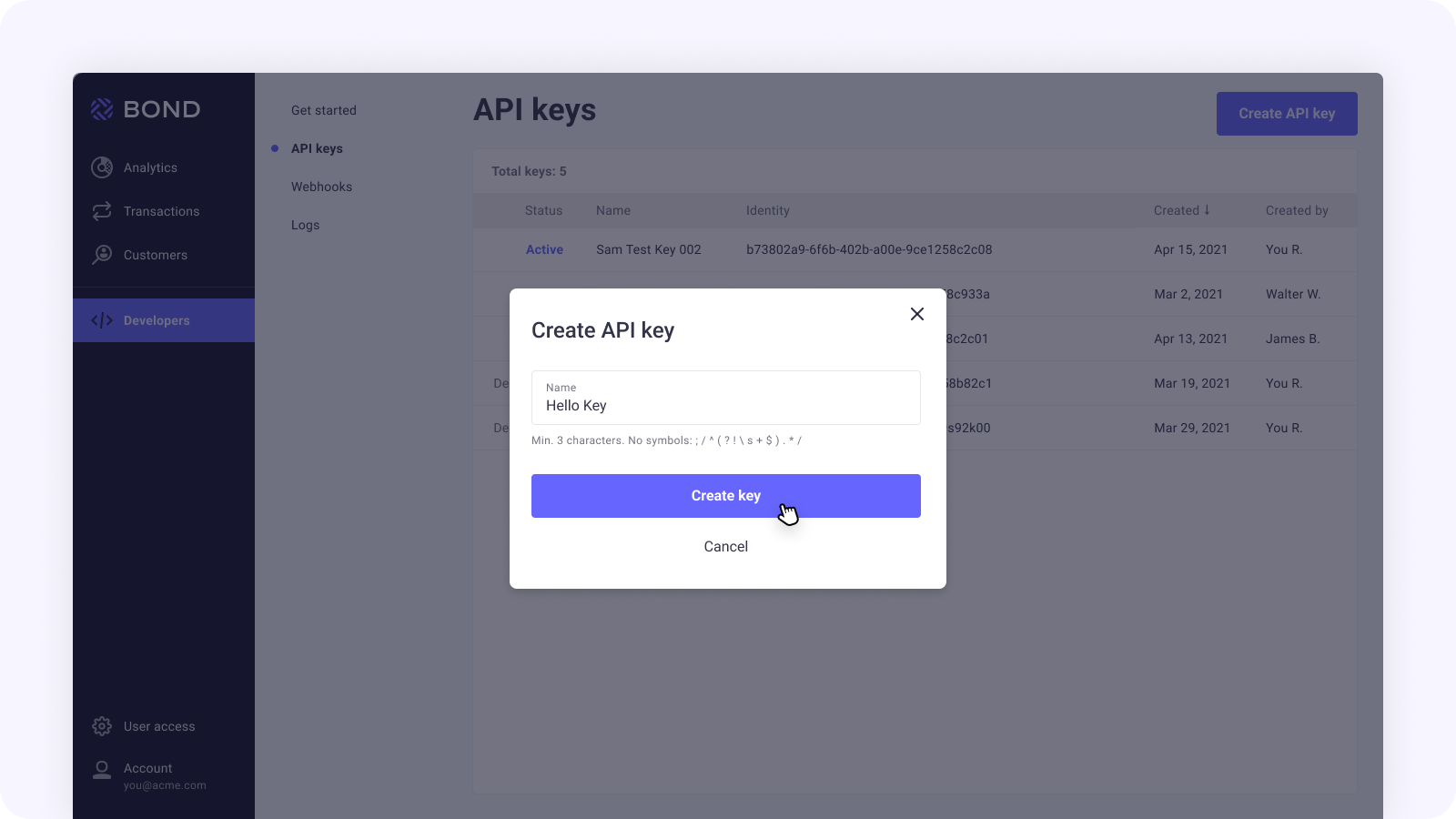
Your new API key is generated and the identity and authorization are displayed.
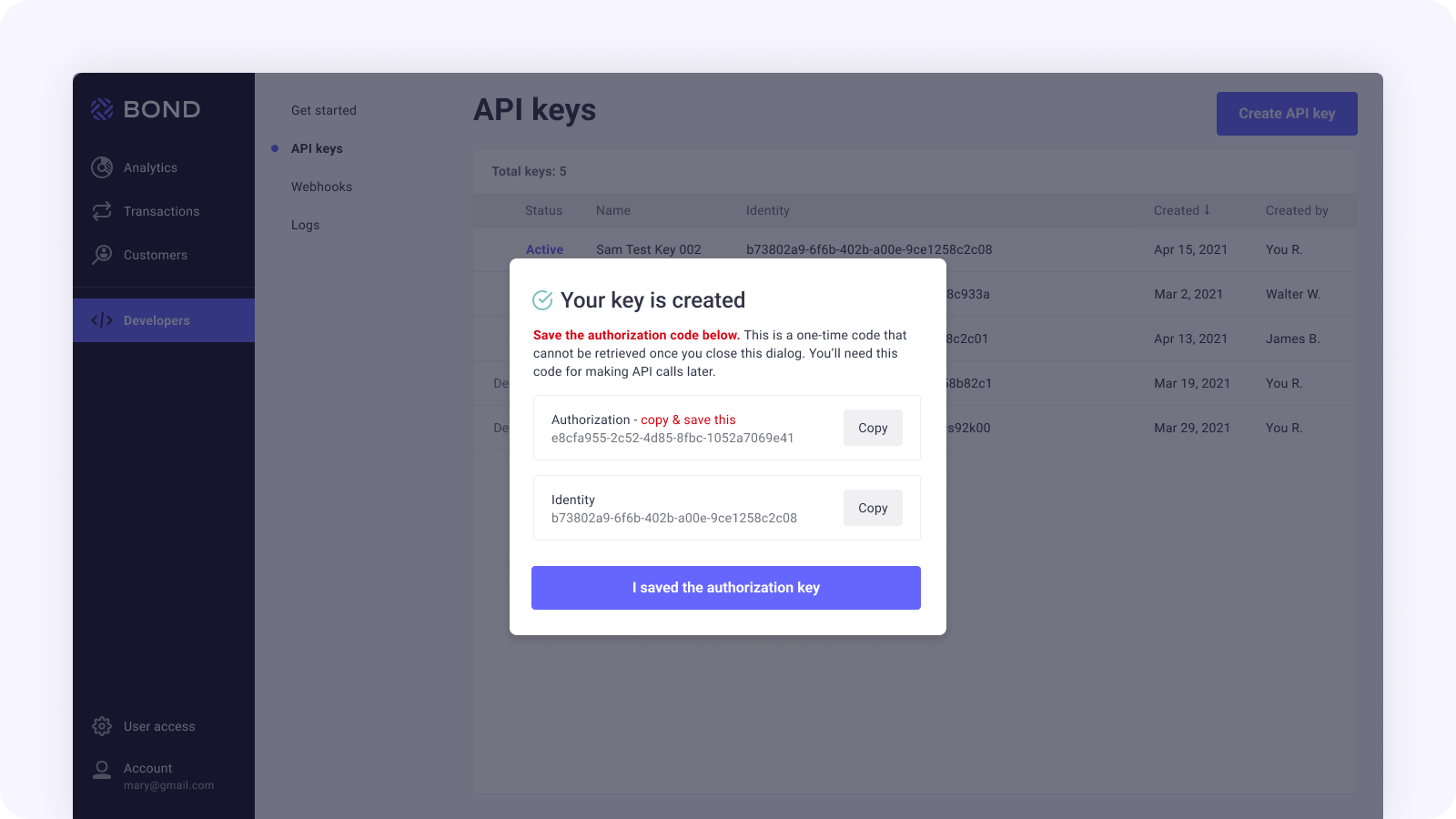
Save a copy of the identity and authorization
If you lose the authorization it can't be retrieved. Make sure to store a copy in a safe place.
You can view the details of your API key, (apart from the authorization) in the API keys page.
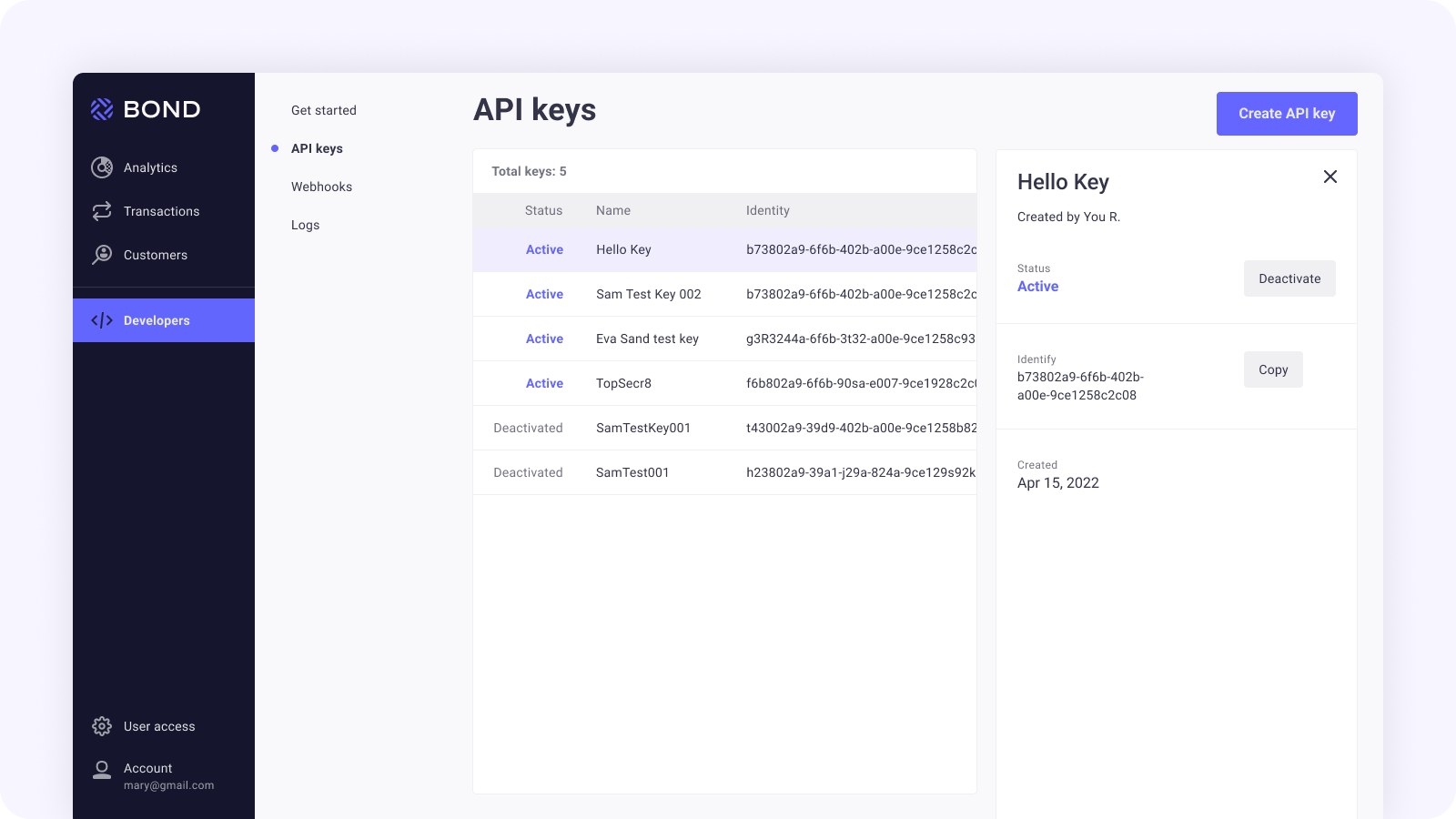
To verify your API key, send the HTTP request show below to the auth
endpoint.
Include your identity and authorization information in the request header.
curl --location --request GET 'https://sandbox.bond.tech/api/v0.1/auth/key/YOUR-IDENTITY' \
--header 'Identity: YOUR-IDENTITY' \
--header 'Authorization: YOUR-AUTHORIZATION'
import requests
url = "https://sandbox.bond.tech/api/v0.1/auth/key/YOUR-IDENTITY"
payload = {}
headers = {
'Identity': 'YOUR-IDENTITY',
'Authorization': 'YOUR-AUTHORIZATION'
}
response = requests.request("GET", url, headers=headers, data = payload)
require "uri"
require "net/http"
url = URI("https://sandbox.bond.tech/api/v0.1/auth/key/YOUR-IDENTITY")
https = Net::HTTP.new(url.host, url.port);
https.use_ssl = true
request = Net::HTTP::Get.new(url)
request["Identity"] = "YOUR-IDENTITY"
request["Authorization"] = "YOUR-AUTHORIZATION"
response = https.request(request)
const data = null;
const xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("GET", "https://sandbox.bond.tech/api/v0.1/auth/key/YOUR-IDENTITY");
xhr.setRequestHeader("Identity", YOUR-IDENTITY);
xhr.setRequestHeader("Authorization", YOUR-AUTHORIZATION);
xhr.send(data);
var client = new RestClient("https://sandbox.bond.tech/api/v0.1/auth/key/YOUR-IDENTITY");
var request = new RestRequest(Method.GET);
request.AddHeader("identity", YOUR-IDENTITY);
request.AddHeader("authorization", YOUR-AUTHORIZATION);
IRestResponse response = client.Execute(request);
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url("https://sandbox.bond.tech/api/v0.1/auth/key/YOUR-IDENTITY")
.get()
.addHeader("identity", YOUR-IDENTITY)
.addHeader("authorization", YOUR-AUTHORIZATION)
.build();
Response response = client.newCall(request).execute();
A successful request returns a 200
response code with your key's non-secret data as shown below.
{
"identity": "de8860e3-5af8-499a-9034-bfe4072d23d4",
"key_name": "starcups",
"role_id": "8a3b727f-c4f1-4b9e-b6ba-be22a6ce92c8",
"role_name": "OS_FULL_ACCESS",
"org_id": "e0cb163c-51dc-44b0-af3c-5c68341068da",
"grantor": "ec003dc6-ebc5-419e-a14f-fad9cbca0d22",
"grantor_os_user_id": "1445e863-43a9-4408-acf0-158448bfa430",
"grantee": "1442b863-43a9-4848-acf0-158448bfa430",
"date_created": "2021-02-09T08:03:21.777887+00:00",
"date_updated": "2021-02-09T12:52:25.394084+00:00",
"date_revoked": null,
"date_expired": null,
"status": "active",
"call_count": 3,
"last_call": "2021-02-09T12:52:25.392641+00:00",
"last_auth": "S",
"last_used": "2021-02-09T12:52:25.393379+00:00",
"last_method": "unknown",
"last_route": "unknown"
}
Use your API key to authenticate all HTTP requests from your Bond account to the Bond APIs. Include the identity and authorization components of the API key in the request header.
With your API key, here are some of the things that the Bond platform enables you to do.
Updated over 2 years ago