6. Creating a card
Creating a new card for your customer.
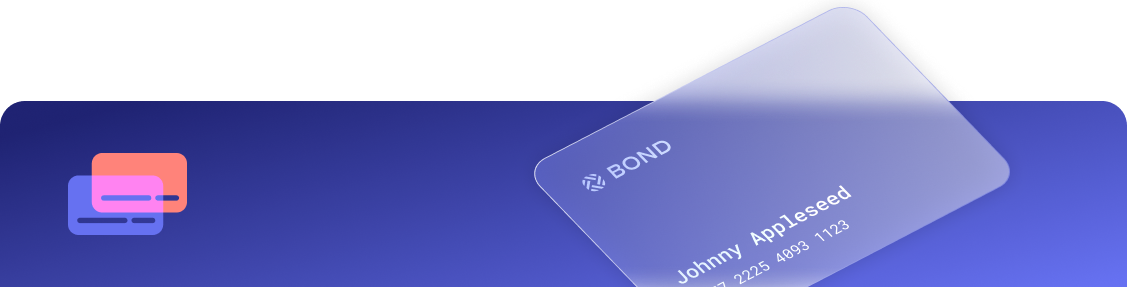
You can create a card for customers who have passed the KYC verification process.
The card contains information relating to the physical or virtual card, while the associated account contains broader information, such as what bank accounts it's connected to.
The card configuration is determined by the program_id
which is provided by the Bond platform based on your requirements. There are three card configurations:
- Virtual
- Physical
- Dual
For details, see Card program ID.
Upon creation, your customer can immediately use the virtual card. If they've been issued with a physical card, they can only use it once it's been activated. See activating a physical card.
The account_id
is based on the program_id
which dictates information such as:
- The KYC provider
- Whether it is a debit/credit program
- The card processor
- The BIN provider
To create a card, use the POST /cards
operation and provide the account_id
as detailed in the cards API.
curl --request POST \
--url https://sandbox.bond.tech/api/v0.1/cards \
--header 'Accept: application/json' \
--header 'Authorization: <YOUR_AUTHORIZATION>' \
--header 'Content-Type: application/json' \
--header 'Identity: <YOUR_IDENTITY>' \
--data '
{
"account_id": "1c667427-5521-4ffd-ac59-16ab73dc133e"
}
'
import requests
url = "https://sandbox.bond.tech/api/v0.1/cards"
payload = {"account_id": "1c667427-5521-4ffd-ac59-16ab73dc133e"}
headers = {
"Accept": "application/json",
"Content-Type": "application/json",
"Identity": "<YOUR_IDENTITY>",
"Authorization": "<YOUR_AUTHORIZATION>"
}
response = requests.post(url, json=payload, headers=headers)
print(response.text)
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://sandbox.bond.tech/api/v0.1/cards")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Accept"] = 'application/json'
request["Content-Type"] = 'application/json'
request["Identity"] = '<YOUR_IDENTITY>'
request["Authorization"] = '<YOUR_AUTHORIZATION>'
request.body = "{\"account_id\":\"1c667427-5521-4ffd-ac59-16ab73dc133e\"}"
response = http.request(request)
puts response.read_bodyconst options = {
method: 'POST',
headers: {
Accept: 'application/json',
'Content-Type': 'application/json',
Identity: '<YOUR_IDENTITY>',
Authorization: '<YOUR_AUTHORIZATION>'
},
body: JSON.stringify({account_id: '1c667427-5521-4ffd-ac59-16ab73dc133e'})
};
fetch('https://sandbox.bond.tech/api/v0.1/cards', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
const options = {
method: 'POST',
headers: {
Accept: 'application/json',
'Content-Type': 'application/json',
Identity: '<YOUR_IDENTITY>',
Authorization: '<YOUR_AUTHORIZATION>'
},
body: JSON.stringify({account_id: '1c667427-5521-4ffd-ac59-16ab73dc133e'})
};
fetch('https://sandbox.bond.tech/api/v0.1/cards', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
var client = new RestClient("https://sandbox.bond.tech/api/v0.1/cards");
var request = new RestRequest(Method.POST);
request.AddHeader("Accept", "application/json");
request.AddHeader("Content-Type", "application/json");
request.AddHeader("Identity", "<YOUR_IDENTITY>");
request.AddHeader("Authorization", "<YOUR_AUTHORIZATION>");
request.AddParameter("application/json", "{\"account_id\":\"1c667427-5521-4ffd-ac59-16ab73dc133e\"}", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
OkHttpClient client = new OkHttpClient();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\"account_id\":\"1c667427-5521-4ffd-ac59-16ab73dc133e\"}");
Request request = new Request.Builder()
.url("https://sandbox.bond.tech/api/v0.1/cards")
.post(body)
.addHeader("Accept", "application/json")
.addHeader("Content-Type", "application/json")
.addHeader("Identity", "<YOUR_IDENTITY>")
.addHeader("Authorization", "<YOUR_AUTHORIZATION>")
.build();
Response response = client.newCall(request).execute();
A successful response returns the card_id
and card_account_id
similar to the example shown below.
{
"card_id": "057c6074-a02d-4a5a-bad9-bbc64b047df7",
"date_updated": "2020-08-16T19:39:34Z",
"date_created": "2020-08-15T19:39:34Z",
"customer_id": "c8940088-21e8-451c-987c-0a0398db3ee5",
"account_id": "26bdeb70-157c-4c44-a04a-3727793b9779",
"expiry_date": "tok_live_7g3eARzeEBJw89rJRCHqHv",
"last_four": "6270",
"card_number": "tok_live_q7kwTYb5YCYznpgRHHTs9p",
"cvv": "tok_live_c94od3AsFWYQ1ecaaMYtFU",
"status": "active",
"card_design_id": null
}
Updated about 2 months ago