How KYB works
Overview and how you can run the Know Your Business verification process for a business, and what webhook events are generated.
Overview
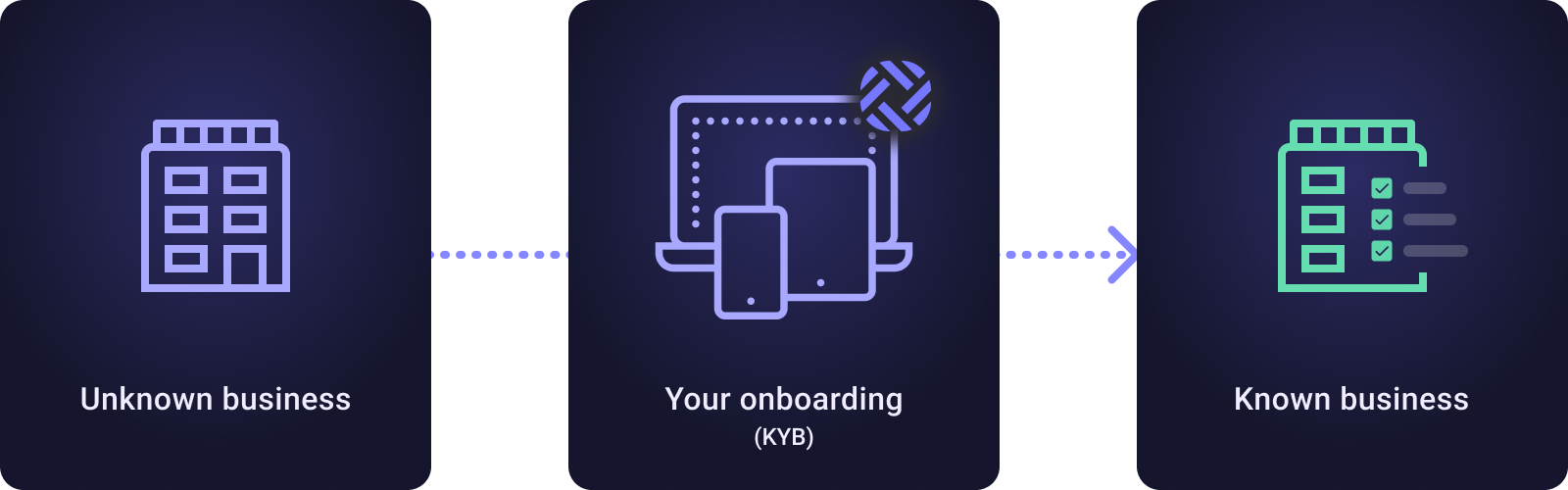
After your business has provided the information necessary to create a Business resource and you have added any beneficial owners, you need to authenticate and verify that information. Using the Business
object, you can perform a number of checks and screenings to ensure compliance. This process is controlled the Bond platforms's KYB service.
This process is a critical step where you validate the identity of your business and its documents to ensure that they're compliant with federal regulations.
Requirement
Before running KYB, make sure that the business resource contains
ein
andphone
values and that these are correct.
See Retrieving a business to view theein
andphone
status, and Updating a business to modify them if necessary.
Running the KYB process
To start the KYB process, use the POST /businesses/{business_id}/verification-kyb
operation with no further parameters.
An example of a request to initiate a KYB process for the business ID 002e0f0e-e39d-4351-876c-afcad30d9c37
is shown below.
curl --request POST \
--url https://sandbox.bond.tech/api/v0.1/businesses/002e0f0e-e39d-4351-876c-afcad30d9c37/verification-kyb \
--header 'Authorization: YOUR-AUTHORIZATION' \
--header 'Identity: YOUR-IDENTITY'
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://sandbox.bond.tech/api/v0.1/businesses/002e0f0e-e39d-4351-876c-afcad30d9c37/verification-kyb")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Post.new(url)
request["Identity"] = 'YOUR-IDENTITY'
request["Authorization"] = 'YOUR-AUTHORIZATION'
response = http.request(request)
puts response.read_body
const options = {
method: 'POST',
headers: {
Identity: 'YOUR-IDENTITY',
Authorization: 'YOUR-AUTHORIZATION'
}
};
fetch('https://sandbox.bond.tech/api/v0.1/businesses/002e0f0e-e39d-4351-876c-afcad30d9c37/verification-kyb', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
import requests
url = "https://sandbox.bond.tech/api/v0.1/businesses/002e0f0e-e39d-4351-876c-afcad30d9c37/verification-kyb
headers = {
'Identity': YOUR-IDENTITY,
'Authorization': YOUR-AUTHORIZATION,
'Content-Type': 'application/json'
}
response = requests.request("POST", url, headers=headers, data = None)
print(response.text.encode('utf8'))
var client = new RestClient("https://sandbox.bond.tech/api/v0.1/businesses/002e0f0e-e39d-4351-876c-afcad30d9c37/verification-kyb");
var request = new RestRequest(Method.POST);
request.AddHeader("Identity", "YOUR-IDENTITY");
request.AddHeader("Authorization", "YOUR-AUTHORIZATION");
IRestResponse response = client.Execute(request);
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url("https://sandbox.bond.tech/api/v0.1/businesses/002e0f0e-e39d-4351-876c-afcad30d9c37/verification-kyb")
.post(null)
.addHeader("Identity", "YOUR-IDENTITY")
.addHeader("Authorization", "YOUR-AUTHORIZATION")
.build();
Response response = client.newCall(request).execute();
When you initiate a KYB process, the KYB service responds with an initiated
message similar to the one shown below.
{
"business_id": "002e0f0e-e39d-4351-876c-afcad30d9c37",
"kyb_status": "initiated"
}
The results of the KYB are sent via a webhook.
If a business fails the KYB check and they can't pass a manual check, you can't issue them a card.
For a complete specification and interactive examples, see Running KYB in the Bond API Reference.
KYB webhook events
Possible responses to a KYB request are shown in the following table.
Event enum values | Description |
---|---|
kyb.verification.approved | KYB passed. |
kyb.verification.error | KYB failed due to a server error. To retry, send a POST /businesses/{business_id}/verification-kyb . |
kyb.verification.initiated | The KYB process has been started. |
kyb.verification.rejected | KYB failed due to low confidence in identity validation. |
kyb.verification.warning | KYB requires manual review. The response contains any business information which could not be verified. |
Possible responses to a KYB check are shown below.
{
"event": "kyb.verification.approved",
"business_id": "002e0f0e-e39d-4351-876c-afcad30d9c37",
"occurred_at": "2021-02-02-00:50:58.484840+00:00"
}
{
"event": "kyb.verification.rejected",
"business_id": "002e0f0e-e39d-4351-876c-afcad30d9c37",
"occurred_at": "2021-02-02-00:50:58.484840+00:00",
"details" :[
{
"code": "rejected_address",
"reason" : "address verification failed"
},
{
"code": "rejected_tin",
"reason" : "TIN verification failed"
},
{
"code": "rejected_name",
"reason" : "name verification failed"
},
{
"code": "rejected_watchlist",
"reason" : "watchlist verification failed."
}
]
}
{
"event":"kyb.verification.warning",
"business_id":"002e0f0e-e39d-4351-876c-afcad30d9c37",
"occurred_at":"2021-02-02-00:50:58.484840+00:00"
}
{
"event": "kyb.verification.error",
"business_id": "002e0f0e-e39d-4351-876c-afcad30d9c37",
"occurred_at": "2021-02-02-00:50:58.484840+00:00"
}
Retrieving the KYB status
To retrieve the KYB status of a business, use the GET /businesses/{business_id}/verification-kyb
operation with no further parameters.
An example of a KYB status retrieval request is shown below.
curl --request GET \
--url https://sandbox.bond.tech/api/v0.1/businesses/002e0f0e-e39d-4351-876c-afcad30d9c37/verification-kyb \
--header 'Authorization: YOUR-AUTHORIZATION' \
--header 'Identity: YOUR-IDENTITY'
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://sandbox.bond.tech/api/v0.1/businesses/002e0f0e-e39d-4351-876c-afcad30d9c37/verification-kyb")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
request = Net::HTTP::Get.new(url)
request["Identity"] = 'YOUR-IDENTITY'
request["Authorization"] = 'YOUR-AUTHORIZATION'
response = http.request(request)
puts response.read_body
const options = {
method: 'GET',
headers: {
Identity: 'YOUR-IDENTITY',
Authorization: 'YOUR-AUTHORIZATION'
}
};
fetch('https://sandbox.bond.tech/api/v0.1/businesses/002e0f0e-e39d-4351-876c-afcad30d9c37/verification-kyb', options)
.then(response => response.json())
.then(response => console.log(response))
.catch(err => console.error(err));
import requests
url = "https://sandbox.bond.tech/api/v0.1/businesses/002e0f0e-e39d-4351-876c-afcad30d9c37/verification-kyb"
headers = {
'Identity': YOUR-IDENTITY,
'Authorization': YOUR-AUTHORIZATION,
'Content-Type': 'application/json'
}
response = requests.request("GET", url, headers=headers)
print(response.text.encode('utf8'))
import requests
url = "https://sandbox.bond.tech/api/v0.1/businesses/002e0f0e-e39d-4351-876c-afcad30d9c37/verification-kyb"
headers = {
"Identity": "YOUR-IDENTITY",
"Authorization": "YOUR-AUTHORIZATION"
}
response = requests.request("GET", url, headers=headers)
print(response.text)
OkHttpClient client = new OkHttpClient();
Request request = new Request.Builder()
.url("https://sandbox.bond.tech/api/v0.1/businesses/002e0f0e-e39d-4351-876c-afcad30d9c37/verification-kyb")
.get()
.addHeader("Identity", "YOUR-IDENTITY")
.addHeader("Authorization", "YOUR-AUTHORIZATION")
.build();
Response response = client.newCall(request).execute();
{
"business_id": "002e0f0e-e39d-4351-876c-afcad30d9c37",
"kyb_status": "rejected",
"details" :
[
{
"code": "rejected_address",
"reason" : "address verification failed"
},
{
"code": "rejected_tin",
"reason" : "TIN verification failed"
},
{
"code": "rejected_name",
"reason" : "name verification failed"
},
{
"code": "rejected_watchlist",
"reason" : "watchlist verification failed."
}
]
}
{
"business_id": "002e0f0e-e39d-4351-876c-afcad30d9c37",
"kyb_status": "approved",
}
For a complete specification and interactive examples, see Retrieving the KYB status in the Bond API Reference.
Updated over 1 year ago