5. Creating a card
Creating a new card for your customer.
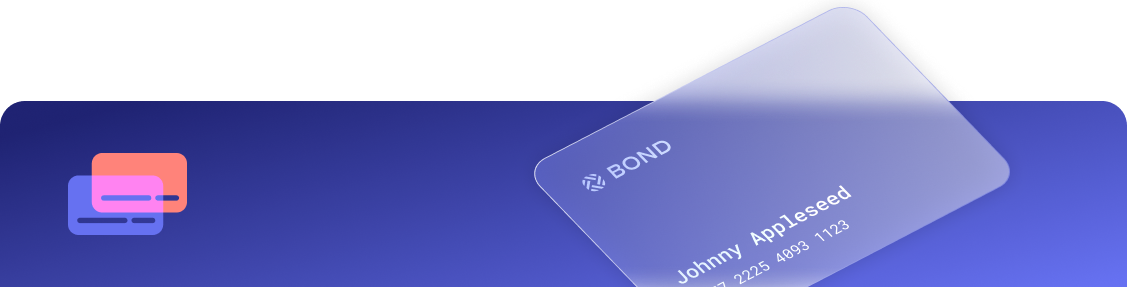
You can create a debit or a credit card for customers who have passed KYC verification.
When a new card is created, you will notice that two references for that card are automatically generated: a card ID and a card account ID. These references are closely related, but are used to manage different types of information about the card.
The card ID is used to reference information about the actual card. You would use the card ID, for instance, to manage information such as:
- Card status (active, stolen, lost)
- Last four digits of the card
- Expiry data
- Cardholder name
The card account ID is an account ID that refers to the bank account to which the card is linked. The card account ID would be used to manage information such as:
- Total number of transactions with the card
- Available balance
- Current balance
- Bank account type
Use the card ID for information related to the actual card, and the card account ID for information about the linked bank account for the card.
Card configuration
When you make the API request to create the card, you must specify the program_id
provided to you by the Bond platform. This will determine whether the card is a debit or credit card, as well as the card configuration.
The card configuration specifies whether the card can be used physically, virtually, or in both ways by the cardholder. It is determined by the program_id
which is provided by the Bond platform based on your product's requirements.
There are three card configurations:
- Physical
- Virtual
- Dual
Physical cards will be issued and shipped to customers, and must be activated before they can be used. Virtual cards live inside a customer's mobile wallet and work only for digital purchases. Dual cards involve a physical card and a virtual card that are issued together, and thus work for both point-of-sale (POS) and digital purchases.
For more details on card configurations, see Card program ID.
Creating a card and card account
A card and card account are simultaneously created from a single POST /cards
request that must contain both the program_id
and customer_id
(or business_id
for commercial cards) in the request body.
The program ID references the details of your card program with the Bond platform. program_id
is a primary key reference to all information related to your brand’s card program. It is visible in Bond Portal and contains information such as:
- The KYC provider
- Whether it is a debit/credit program
- The card processor
- The BIN provider
The customer_id refers to the intended cardholder's representation within the Bond platform. The business_id refers to a business associated with the intended cardholder and is used instead of customer_id
for commercial cards.
To create a card and card account, use the POST /cards
operation and provide the program_id
and customer_id
as detailed in the cards API. To create a commercial card, you must provide the program_id
and business_id
.
curl --request POST \
--url https://sandbox.bond.tech/api/v0/cards \
--header 'Identity: YOUR-IDENTITY' \
--header 'Authorization: YOUR-AUTHORIZATION' \
--header 'Content-Type: application/json' \
--data '{"customer_id":"094cd49b-6412-429f-a396-314097a6c3b9",
"program_id":"2742ff6a-7455-4066-8b45-ae12d3acca34"}'
import requests
url = "https://sandbox.bond.tech/api/v0/core/cards"
payload = {
"customer_id":"094cd49b-6412-429f-a396-314097a6c3b9",
"program_id":"2742ff6a-7455-4066-8b45-ae12d3acca34"
}
headers = {
"Content-Type": "application/json",
"Identity": YOUR-IDENTITY,
"Authorization": YOUR-AUTHORIZATION
}
response = requests.request("POST", url, json=payload, headers=headers)
print(response.text)
require 'uri'
require 'net/http'
require 'openssl'
url = URI("https://sandbox.bond.tech/api/v0/core/cards")
http = Net::HTTP.new(url.host, url.port)
http.use_ssl = true
http.verify_mode = OpenSSL::SSL::VERIFY_NONE
request = Net::HTTP::Post.new(url)
request["Content-Type"] = 'application/json'
request["Identity"] = YOUR-IDENTITY
request["Authorization"] = YOUR-AUTHORIZATION
request.body = "{\"customer_id\":\"094cd49b-6412-429f-a396-314097a6c3b9\",\"program_id\":\"2742ff6a-7455-4066-8b45-ae12d3acca34\"}"
response = http.request(request)
puts response.read_body
const data = JSON.stringify({
"customer_id":"094cd49b-6412-429f-a396-314097a6c3b9",
"program_id":"2742ff6a-7455-4066-8b45-ae12d3acca34"
});
const xhr = new XMLHttpRequest();
xhr.addEventListener("readystatechange", function () {
if (this.readyState === this.DONE) {
console.log(this.responseText);
}
});
xhr.open("POST", "https://sandbox.bond.tech/api/v0/core/cards");
xhr.setRequestHeader("Content-Type", "application/json");
xhr.setRequestHeader("Identity", YOUR-IDENTITY);
xhr.setRequestHeader("Authorization", YOUR-AUTHORIZATION);
xhr.send(data);
var client = new RestClient("https://sandbox.bond.tech/api/v0/core/cards");
var request = new RestRequest(Method.POST);
request.AddHeader("Content-Type", "application/json");
request.AddHeader("Identity", YOUR-IDENTITY);
request.AddHeader("Authorization", YOUR-AUTHORIZATION);
request.AddParameter("application/json", "{\"customer_id\":\"094cd49b-6412-429f-a396-314097a6c3b9\",\"program_id\":\"2742ff6a-7455-4066-8b45-ae12d3acca34\"}", ParameterType.RequestBody);
IRestResponse response = client.Execute(request);
OkHttpClient client = new OkHttpClient();
MediaType mediaType = MediaType.parse("application/json");
RequestBody body = RequestBody.create(mediaType, "{\"customer_id\":\"094cd49b-6412-429f-a396-314097a6c3b9\",\"program_id\":\"2742ff6a-7455-4066-8b45-ae12d3acca34\"}");
Request request = new Request.Builder()
.url("https://sandbox.bond.tech/api/v0/core/cards")
.post(body)
.addHeader("Content-Type", "application/json")
.addHeader("Identity", YOUR-IDENTITY)
.addHeader("Authorization", YOUR-AUTHORIZATION)
.build();
Response response = client.newCall(request).execute();
A successful 201 response contains the card's card_id
and card_account_id
, as shown in the example below:
{
"card_id": "6dd74dde-8114-4a61-b795-114946984345",
"last_four": "9655",
"status": "Active",
"pseudo_dda": "99947206486601",
"sponsor_bank_routing_number": "101115315",
"card_design_id": "null",
"program_id": "97e915e1-43e1-4516-89e8-577620c006cb",
"date_created": "2021-12-02T01:16:24.962390+00:00",
"card_account_id": "9c7549dc-2d64-4c14-8842-d1f8ade38d6c",
"customer_id": "fd644b55-c90c-4301-9e7b-e56383d1456e"
}
Card activation after creation
For physical card configurations, your customer will be issued a physical card and can only use it once it has been activated. See activating a physical card for more details.
For virtual card configurations, your customer can immediately use the card once it has been created.
For dual card configurations, the virtual card and physical card will be issued together and will have the same card number but different CVVs or expiry dates. Once you activate the physical card, the card number, expiry, and CVV combination of the virtual card will no longer be valid. However, if the card has been added to a mobile wallet, the tokenized card information in the mobile wallet card will be updated to match the physical card details automatically without user intervention, and the BondCards SDK will automatically start showing the card information of the physical card. The cardholder experience will be that once they activate their physical card in your app, they’ll be able to continue using their card in their mobile wallet as expected.
Updated over 2 years ago